Builder Docs
Fundamentals for advanced building on NEAR
We're building resources for technical founders, indie hackers, and hobbyists on NEAR. The aim is to explain foundational concepts, how NEAR is differentiated from other blockchains, and "how to think" as a builder.
Core concepts include:
Asynchronous transactions
Blockchain transactions are verified by the protocol, by proof-of-stake validators running nearcore, then turned into "receipt" objects.
These receipts will find their way to the correct shard, where the target smart contract is being called. Each named account, like root.near has a dedicated state key where a smart contract is stored. In that sense, a named account is being called, and the contract state is loaded into the appropriate runtime.
A helpful adjustment in perspective is to consider how your smart contracts handle cross-contract calls. This doesn't add much complexity, but is important to consider if the response from your cross-contract call needs to be matched with the promise index, and any relevant state needed to continue operation.
Account model
The protocol has named accounts (similar to Ethereum Name Service) built in. Named accounts behave similar to internet DNS, in that the owner of example.near can create my_app-123.example.near, and it can be initialized with any public key.
Implicit accounts are the only other type of account, and they are the most minimal entity necessary, and do not have a slot for a smart contract. Implicit account names look like common 0x addresses across ecosystems.
Account key pairs
Both secp256k1 and ed25519 key pair types are supported. The former is used by Ethereum, and the latter by Solana. Each named account can have multiple keys, of either curve type. Among other things, this means key rotation is built into the protocol.
A key pair type is used to sign transactions, and there are two kinds:
- Full access key: authorized to execute any of the NEAR actions. (FunctionCall, CreateAccount, RemoveKey, etc.)
- Limited access key: authorized to only use the FunctionCall Action, without the ability to attach a deposit. These access keys can be scoped to include all contract method calls, or limited to a subset. Upon creation, they can be given an allowance of NEAR, that can only be consumed via function-call execution, calculated by the gas price at the time.
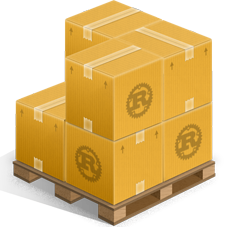
Smart contracts
NEAR supports smart contracts written in different languages. These docs will focus on Rust smart contracts. These are compiled to WebAssembly, using the target wasm32-unknown-unknown, and outputs deterministic code hashes using containers. This optimization is typically done before a production deploy, and can be done with cargo near.
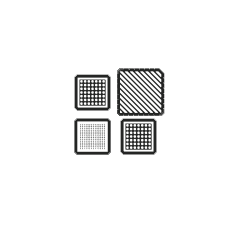
Tracking transactions
NEAR is a sharded blockchain, but it doesn't increase the difficulty of tracking transactions. There are two types of transaction finality: optimistic and final. Transactions also have a status that can be queried by RPC calls, or subscribed to via event listeners. Pending, Included, and Executed are examples of tx statuses.
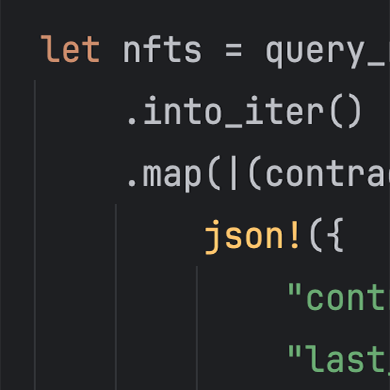
Brief code snippets
We'll be populating this resource with dead-simple snippets/examples, for reference and reminders. Candidate topics include:
- Simple Rust daemon interacting with NEAR
- Simple JS/TS service
- Indexing fundamentals
- Customizing a validator's staking pool contract
- Cross-contract calls that saves and references state by promise index
- Killer yield/resume example
- Running your own RPC